This is part 1 of the "Web client communication to Unreal and back" series.
Before we start trying to send messages back and forth between the web client and the streaming game package, we will make a simple stateful control in a fresh web client.
Please make sure you have read the following article prior to proceeding.
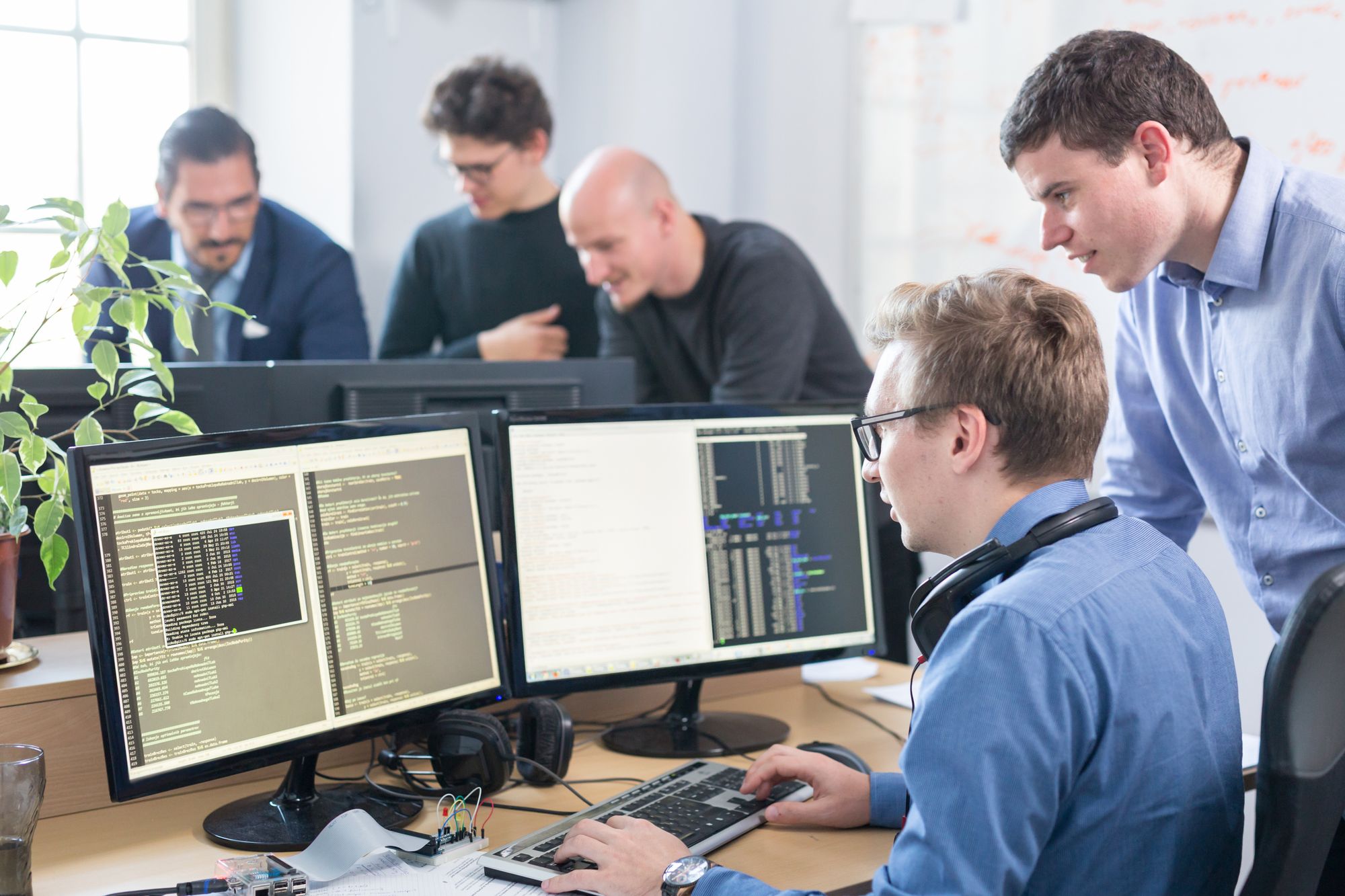
We are going to create a button that is similar to the fullscreen button in the top right corner of the web client template. The web client will remember its state of TRUE or FALSE and change the icon of the new button based on that value.
Define the Button
Once you have downloaded a new copy of the web client, open the App.tsx file in your code editor of choice. Around line 160, find the declaration for the EmbeddedView, the first line of which looks something like this:
const EmbeddedView: React.FC<ViewProps> = (props: ViewProps) => {
We are going to add another button to the UI. Just before the StreamerStatus declaration, add the following code:
<Button
onClick={() => toggleLight(!lightIsOn)}
style={{ position: 'absolute', top: 50, right: 10 }}>
<Icon name={lightIsOn ? "toggle on" : "toggle off"} />
</Button>
So what’s happening here?
- The onClick handler toggleLight has not been defined yet, but we will address that in a moment.
- The position "top: 50" places the new button directly underneath the fullscreen button.
- The icon checks a lightIsOn property to decide whether to use the toggle on or toggle off icon from the https://react.semantic-ui.com/elements/icon/ library.
If we try to run the web client now, it won’t do anything yet. Let’s add the onClick handler and finish up part 1.
Add the ClickHandler
At the top of the EmbeddedView, after the other const declarations, type or paste this line:
const [lightIsOn, toggleLight] = useState(false);
This gives us a new property called lightIsOn and a function called toggleLight. useState(false) sets the lightIsOn property to false when it’s declared.
Looking back at the button definition created earlier, the onClick handler calls toggleLight function and sets lightIsOn to whatever the opposite value is. So if the button is clicked when lightIsOn is FALSE, lightIsOn gets changed to TRUE, and vice versa.
In the Icon name section, we check the value of lightIsOn; if it is TRUE the web client displays the toggle on icon, if it is FALSE, the toggle off button is rendered instead.
Go to Part 2!