To use the Pre-Launch functionality in your custom client, you must upgrade the client to the new 5.0.1 SDK version. Once upgraded, follow these steps to integrate Pre-Launch into your application.
Updating the Launch Request Hook
In your main App.tsx
file, where you issue a launch request, import the additional preLaunchRequest
hook:
const [status, launchRequest, queueLaunchRequest, resetLaunchRequest, preLaunchRequest] = useLaunchRequest(
platform,
modelDefinition,
launchRequestOptions
);
Adding the Pre-Launch Effect
Next, add the following useEffect
section to handle the pre-launch request:
useEffect(() => {
const handlePreLaunchRequest = async () => {
if (modelDefinition && modelDefinition.type !== ModelDefinitionType.Undefined) {
if (clientOptions.LaunchType !== 'local') {
try {
await preLaunchRequest(20000);
} catch (err) {
setLaunchRequestError(err);
}
}
}
};
handlePreLaunchRequest();
}, [modelDefinition, preLaunchRequest]);
How Pre-Launch Works
You do not need to modify any other part of your custom client code.
The Pre-Launch request is useful if you want to start a stream in the background and consume it in response to a user interaction. This can significantly reduce the perceived launch time, improving user experience.
Key considerations:
- The
preLaunchRequest(20000)
parameter is in milliseconds. This specifies how long the pre-launched session remains active. - The maximum allowed pre-launch duration is 5 minutes.
- If the user does not start the session within the specified time, the pre-launched session will terminate.
- If the user starts the session after the pre-launch duration has expired, the launch request will follow the normal route.
- If the user starts the session within the pre-launch window, the time to the first frame will be significantly faster.
- If the user requests the stream before it is ready, the system will attempt to join the background launch request in progress, reducing perceived wait time.
Step 4: Reset to Launch View on Session Completion
In the App.tsx
, monitor the stream status, and reset to initial launch view when the session ends StreamerStatus.Completed
.
if (streamerStatus === StreamerStatus.Completed) {
return <LaunchView Launch={launch} />;
}
Important Notes
- Since the pre-launch functionality keeps the game in a running state, you must ensure the game can wait for the client to connect if needed.
- If your game requires the client to send an initial message before proceeding, you are responsible for implementing a suspension mode that waits for the client’s connection.
- For details on implementing messaging between the client and the game, refer to the following articles:
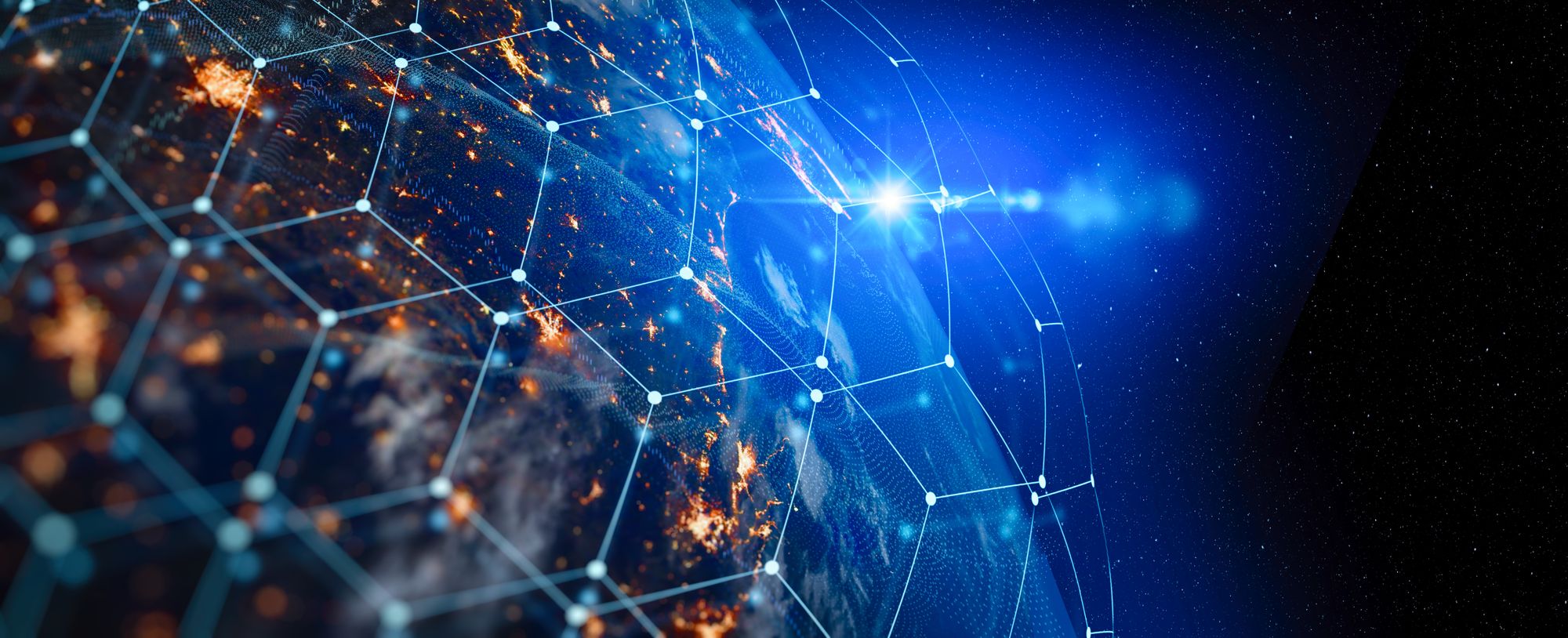
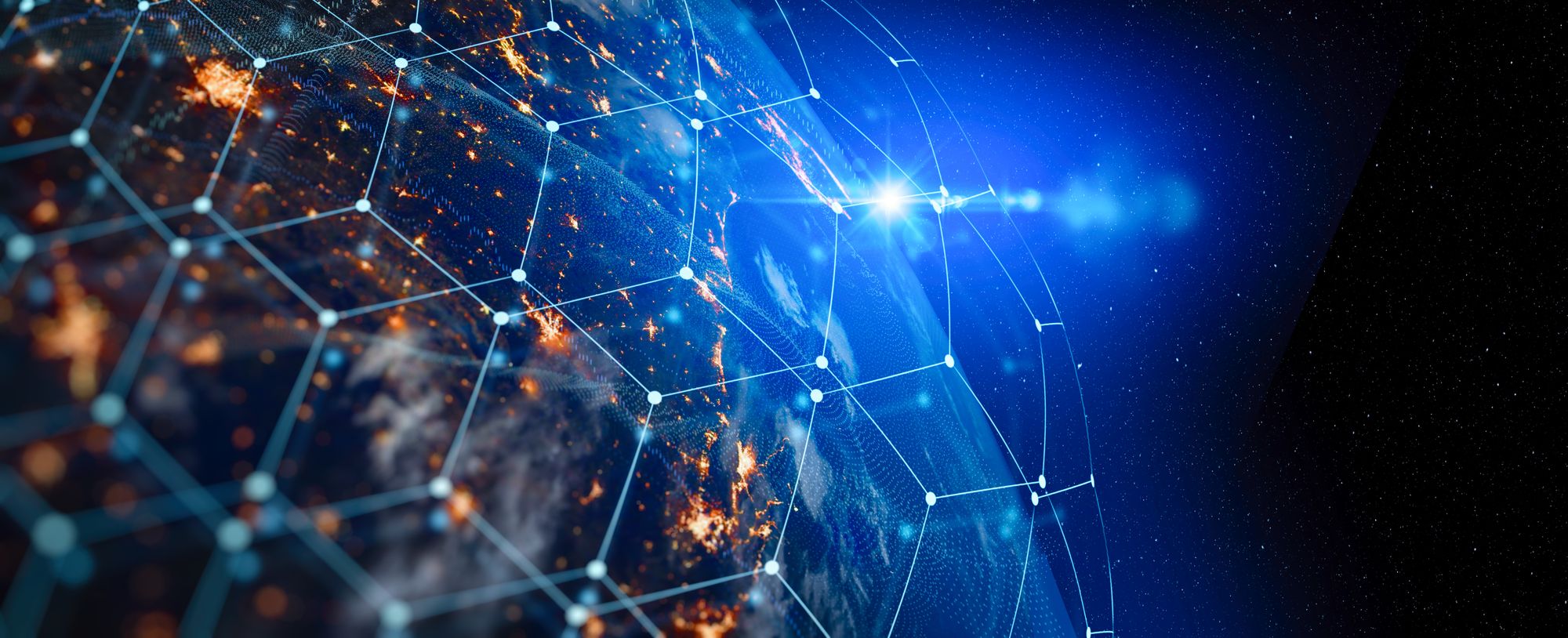