What is Collaboration?
This is a way to use a single session but have multiple streams (i.e.: collaborators). The maximum number of supported collaborators will be between 2 - 6, as each added stream increases consumption of server resources.
In some cases, particularly in the testing phase, you may want to share your 3D streaming project with various collaborators and stakeholders. This is built in to the preview client, but some modifications are required to enable it in your custom web client.
When the end user requests a streaming session via your web client, the session is intended to be just for their use. This session is like a private room, identified by a dynamically-generated environmentId. If you generate a URL containing that unique environmentId, you can use it to share your session with one or more collaborators.
When a collaborator connects to the session they will be able to control and interact with the model. Everyone connected in this way can see and perform these interactions.
Enable Unrestricted Access
For the collaboration feature to work, unrestricted access has to be enabled in the Project Settings window in the PureWeb Console.
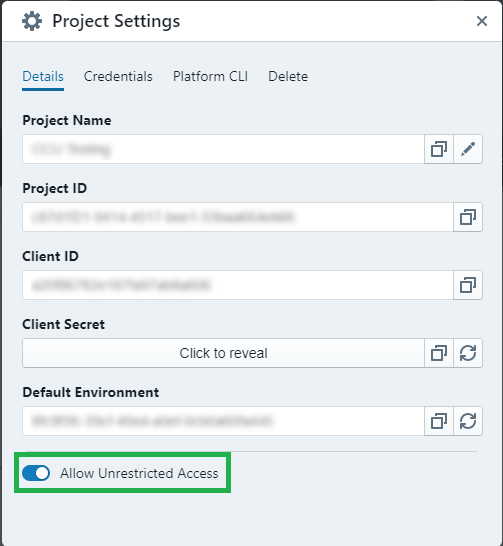
Manually test in the Preview client (optional)
Let's test collaboration using the PureWeb preview client first.
From your console account, click on the "Preview" button on the model tile you want to collaborate on. This will open a new tab with the Preview client and an appropriate link to launch a model stream.
In the new tab, open the web browser's Console tab (in Chrome the hotkey is CTRL+SHIFT+J). There will be a message near the top that starts with "[client] Environment id: " followed by an id string. This is the unique environmentId of the session, and will be different each time (i.e.: if you reloaded the page you'd get a different ID).
We're going to use it in a moment though, so note it down somewhere.
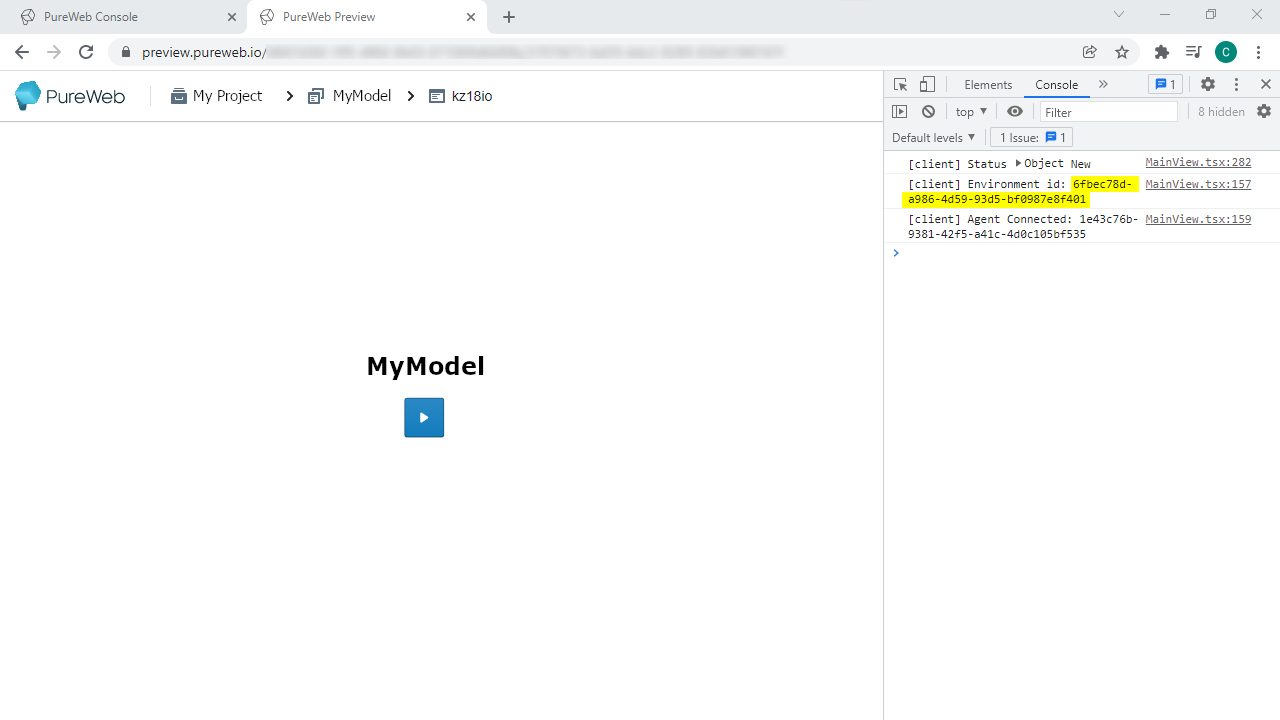
Copy the full URL from the the address bar, then open a new browser tab or window. In the address bar of the new window, paste the copied URL. Next, add two additional parameters (environmentId and collaboration) at the end following a question mark, filling out the appropriate values after the equals sign for each.
It should look similar to the below:
preview.pureweb.io/hash/version?environmentId=copiedEnvironmentId&collaboration=true
When I click play in this new browser tab, the interactions with the model can be seen in both windows. You could now send this link to someone else, and both people would be able interact with the model and see the results.
Next let's build something like this into our custom web client!
Create a collaborator link in your web client
For context, let's look at where a session gets created in the web client. Open the App.tsx file of your web client.
Around line 241 is the code where a session gets created. When requesting a session via your web client, clientOptions.EnvironmentId is empty and an environment is being created for the new session. It looks like this:
await platform.useAnonymousCredentials(clientOptions.ProjectId, clientOptions.EnvironmentId);
After the session has been created, you can then access platform.credentials.agent.environmentId to retrieve the environment ID the current session is running from and generate a collaborator link.
Generate the collaborator link in a function
We'll make a very simple function to do this, then connect it to a button that will display the collaborator link in an alert window.
Still in the App.tsx file, find the definition for the EmbeddedView (around line 155), which looks like this:
const EmbeddedView: React.FC<ViewProps> = (props: ViewProps) => {
Directly following the const declarations, create a new function named getCollabURL.
function getCollabURL()
{
//only create a URL if we have a valid environment
if (platform.credentials.agent.environmentId) {
//create the collaborator URL
var collabUrl = myUrl + '?environmentId=' + platform.credentials.agent.environmentId + '&collaboration=true';
alert(collabUrl);
};
}
Note that the value of myUrl above should be to the location you have deployed your customized web client. (In this example it is 'http://localhost:3000').
Display the link on screen
A little further down, create another button below the full screen button, around line 198.
<Button
onClick={() => getCollabURL()}
style={{ position: 'absolute', top: 10, right: 80}}>
<Icon name="linkify"/>
</Button>
When the button is clicked, a simple alert window will pop up, containing the collaboration URL we created in the getCollabURL function earlier. Copy this link and send it to your collaborators, or even try it yourself in another browser window!
Rather than the alert message, you'll want to add a control that will display the URL and allow it to be copied more elegantly. A simple text box would suffice, but choose the method that suits your experience.
Joining as a collaborator
When the incoming user opens the link in their browser, they will be connected to the specified environment, which will allow each user to interact, and see the other's interactions.
Here's a quick video demo showing testing this in a web client in a local development environment.
Remember that each added stream increases consumption of server resources. As those resources reach their limits, the user experience will begin to degrade or may even become unstable at a certain point. This will depend on the complexity of the game project and its hardware utilization, so it's important to test what number of collaborators your project can handle.